How is Everhood going + Lets create 2D lasers in unity !
Welcome to our sixth devlog !
How is Everhood going
We are doing very good progress on Everhood! Chris is creating more and more in-game content, no one can stop him! From my side (Jordi), I have been working on fixing and creating new in-game logic, don't want to do spoilers but, I bet you will be amazed :D
As I mentioned in Game Localization devlog, trying to not spoil the story and the in-game content is one of our main goals. Here's all we can say for the moment.
To compensate I've made a second mini tutorial/tip for unity c#, you can check the first tutorial/tip here.
Lets create a simple 2D laser! - Unity C#
Difficulty: Beginner
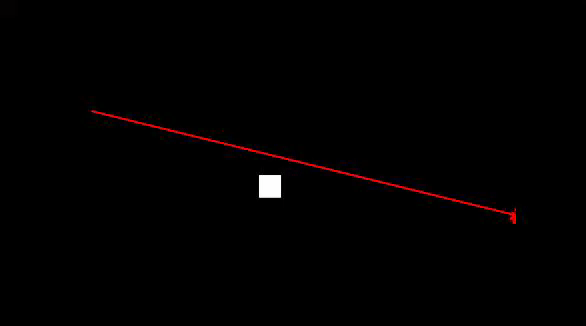
Lasers...who doesn't like lasers?
As I love both lasers and each of you, well, let's create the laser logic step by step!
First, let's create a new empty game object and assign a LineRenderer component to it.
Next, make sure to assign the Sprites-default material and set the alignment to "Transform Z"
Then, create a new script called Laser and open it.
Now let's get the line renderer component at Start().
public class Laser : MonoBehaviour { private LineRenderer _lineRenderer; // Use this for initialization void Start() { _lineRenderer = GetComponent<linerenderer>(); } }
Next, we need to know when an object collides with the laser, let's use Raycast!
public class Laser : MonoBehaviour { .... // Update is called once per frame void Update() { RaycastHit2D hit = Physics2D.Raycast(transform.position, transform.up); if (hit.collider) { } else { } } }
Finally, we need to set line renderer position according to the raycast. Keep in mind that the line renderer have two points 0 and 1, so we need to set both the start point and the end point.
The start point is going to be the main transform position,
The end point is going to be the raycast hit point position,
If the raycast don't hit something, just set the end point to an infinite or large value.
public class Laser : MonoBehaviour { .... // Update is called once per frame void Update() { _lineRenderer.SetPosition(0, transform.position); RaycastHit2D hit = Physics2D.Raycast(transform.position, transform.up); if (hit.collider) { _lineRenderer.SetPosition(1, new Vector3(hit.point.x, hit.point.y, transform.position.z)); } else { _lineRenderer.SetPosition(1, transform.up * 2000); } } }
Assign Laser script to the empty game object we just created, set editor to play mode et voila!
I hope this mini tutorial was useful!
// Jordi
Wishlist us here so you don't miss any news:
Get Everhood
Everhood
Status | Prototype |
Author | EverhoodDev |
Genre | Adventure, Rhythm, Role Playing |
Tags | 2D, Experimental, Music, Mystery, Pixel Art, Top Down Adventure, Undertale |
Languages | English |
More posts
- Everhood is OutMar 07, 2021
- EVERHOOD RELEASE DATE ANNOUCEMENTFeb 01, 2021
- The divine moments of creativityJan 27, 2021
- A look back into the dark timesJan 20, 2021
- Testing, fixing, testing and testingDec 30, 2020
- Hectic weeks and some thoughts!Dec 09, 2020
- Time to start wrapping up the game again!Nov 18, 2020
- Game engine version change and just polish, polish, polishOct 30, 2020
- Lot of work right now!Oct 09, 2020
- Yet another postSep 25, 2020
Comments
Log in with itch.io to leave a comment.
one quetion : how to specify a collider that the lazer should stop on it cuz this work with all collider
btw nice tutorial <3 it
Hey! Glad you liked it. Just add an if statement to check if the tag is equal to "Wall" for example.